Series Spring Boot: Spring Boot 0: Làm chủ Spring Boot, Zero to Hero và Series Spring Boot từ con số 0
Series Spring Core: tại đây
Dưới đây là Series Spring Thymeleaf
- Thymeleaf là gì? So sánh JSP, JSF với Thymeleaf
- Code ví dụ Spring MVC Thymeleaf Hello – dùng XML config
- Code ví dụ Spring MVC Thymeleaf Hello – dùng annotation config
- Submit form với Thymeleaf, Code ví dụ Spring Thymeleaf Form
- Code ví dụ hiển thị List, Set, Map với Thymeleaf
- Code ví dụ đa ngôn ngữ với Thymeleaf Internationalization / i18n
- Code ví dụ gửi email – gmail với Thymeleaf + Spring
Để thực hiện đa ngôn ngữ với Thymleaf, ta chia ngôn ngữ ra các file .properties (mỗi ngôn ngữ 1 file, kết thúc file sẽ là local của ngôn ngữ đó ví dụ vi, us, en…).
Khi chọn hiển thị ngôn ngữ nào, hệ thông sẽ đọc text từ file .properties tương ứng để hiển thị.
Ví dụ:
Tạo file .properties
hello=Xin Chào
Đăng ký file .properties với spring
<bean id="messageSource"
class="org.springframework.context.support.ResourceBundleMessageSource">
<property name="basenames">
<list>
<value>i18n/messages</value>
</list>
</property>
<property name="defaultEncoding" value="UTF-8" />
</bean>
Hiển thị text trên file view:
<p th:text="#{hello}"></p>
Code ví dụ đa ngôn ngữ với Thymeleaf Internationalization i18n
Tạo maven project:
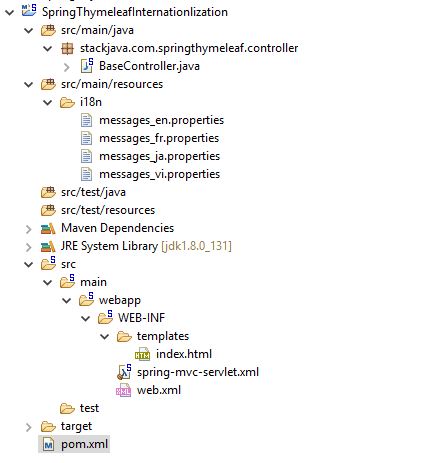
Thư viện sử dụng:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>stackjava.com</groupId>
<artifactId>SpringThymeleaf</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>war</packaging>
<properties>
<spring.version>5.0.2.RELEASE</spring.version>
<thymeleaf.version>3.0.9.RELEASE</thymeleaf.version>
</properties>
<dependencies>
<!-- Spring -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>${spring.version}</version>
</dependency>
<!-- Thymeleaf -->
<dependency>
<groupId>org.thymeleaf</groupId>
<artifactId>thymeleaf</artifactId>
<version>${thymeleaf.version}</version>
</dependency>
<dependency>
<groupId>org.thymeleaf</groupId>
<artifactId>thymeleaf-spring5</artifactId>
<version>${thymeleaf.version}</version>
</dependency>
<!-- javax.servlet-api -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>4.0.0</version>
<scope>provided</scope>
</dependency>
</dependencies>
</project>
File Spring config
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-4.3.xsd
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd">
<context:component-scan base-package="stackjava.com.springthymeleaf" />
<bean id="templateResolver"
class="org.thymeleaf.spring5.templateresolver.SpringResourceTemplateResolver">
<property name="prefix" value="/WEB-INF/templates/" />
<property name="suffix" value=".html" />
<property name="characterEncoding" value="UTF-8" />
<property name="templateMode" value="HTML5" />
<property name="cacheable" value="false" />
</bean>
<bean id="templateEngine" class="org.thymeleaf.spring5.SpringTemplateEngine">
<property name="templateResolver" ref="templateResolver" />
</bean>
<bean class="org.thymeleaf.spring5.view.ThymeleafViewResolver">
<property name="templateEngine" ref="templateEngine" />
<property name="contentType" value="text/html; charset=UTF-8" />
</bean>
<bean id="messageSource"
class="org.springframework.context.support.ResourceBundleMessageSource">
<property name="basenames">
<list>
<value>i18n/messages</value>
</list>
</property>
<property name="defaultEncoding" value="UTF-8" />
</bean>
<!-- <bean id="messageSource"
class="org.springframework.context.support.ReloadableResourceBundleMessageSource">
<property name="basename" value="i18n/messages" />
<property name="defaultEncoding" value="UTF-8" />
</bean> -->
<!-- <bean id="localeResolver" class="org.springframework.web.servlet.i18n.SessionLocaleResolver"> -->
<bean id="localeResolver"
class="org.springframework.web.servlet.i18n.CookieLocaleResolver">
<property name="defaultLocale" value="en" />
<property name="cookieName" value="myAppLocaleCookie"></property>
<property name="cookieMaxAge" value="3600"></property>
</bean>
<mvc:interceptors>
<bean class="org.springframework.web.servlet.i18n.LocaleChangeInterceptor">
<property name="paramName" value="language" />
</bean>
</mvc:interceptors>
</beans>
- Bean messageSource sẽ thực hiện khai báo các file properties
- trong ví dụ này mình khai báo các file properties nằm ở folder i18n, có tên bắt đầu bằng messages
- nếu bạn sử dụng nhiều file properties ở các folder khác nhau thì có thể thêm nó vào list của thẻ <property name=”basenames”>
- Bean localeResolver sẽ xác định ngôn ngữ hiển thị trên view bằng cách nào, ví dụ thông qua session, qua cookies
- Trong ví dụ này, mình sẽ xác định ngôn ngữ hiển thị qua cookies với biến myAppLocaleCookies lưu trên trình duyệt
- Thẻ <mvc:interceptors> và org.springframework.web.servlet.i18n.LocaleChangeInterceptor sẽ xác định thay đổi ngôn ngữ hiển thị thông qua param name nào trên trình duyệt. Ví dụ trên URL có param: ?language=en thì tiếng anh sẽ được hiển thị
(Xem lại: Hiển thị đa ngôn ngữ với Spring MVC)
File web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
version="2.5">
<display-name>SpringThymeleaf</display-name>
<servlet>
<servlet-name>spring-mvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>spring-mvc</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
</web-app>
File controller
package stackjava.com.springthymeleaf.controller;
import javax.servlet.http.HttpServletRequest;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.MessageSource;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class BaseController {
@Autowired
private MessageSource messageSource;
@RequestMapping("/")
public String index(final Model model, HttpServletRequest request) {
String message = messageSource.getMessage("hello", null, "default message", request.getLocale());
model.addAttribute("message", message);
return "index";
}
}
Ngoài hiển thị đa ngôn ngữ ở view, bạn cũng có thể lấy ngôn ngữ theo locale, locale này có thể thấy từ locale mà request gửi lên hoặc dựa theo thông tin nào đó từ request để xác định request.
Vị dụ mình lấy locale ở request và sử dụng messageSource để lấy message theo ngôn ngữ ở file .properties
Các file .properties:
messages_en.properties
hello=Hello
messages_vi.properties
hello=Xin Chào
messages_vi.properties
hello=Xin Chào
messages_ja.properties
hello=こんにちは
File view:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Spring MVC + Thymeleaf</title>
</head>
<body>
<h1>Spring Thymeleaf Internationalization</h1>
Language :
<a href="?language=en">English</a>|
<a href="?language=vi_VN">Viet Nam</a>|
<a href="?language=ja_JP">Japanese</a>|
<a href="?language=fr_FR">France</a>
<p th:text="${message}">User test</p>
<p th:text="#{hello}"></p><br/>
</body>
</html>
Demo:
Default hiển thị tiếng anh
Hiển thị tiếng Việt
Hiển thị tiếng Nhật
Hiển thị tiếng Pháp
Okay, Done!
Download code ví dụ trên tại đây
Loạt bài chủ đề Java trên trang stackjava.com bản quyền thuộc thầy Trần Hữu Cương. Bài viết đăng trên blog Techmaster được sự đồng ý của tác giả.
Thầy Trần Hữu Cương đã và đang tham gia giảng dạy tại Techmater khoá Lộ trình Java Spring Boot Full Stack
Link gốc bài viết tại đây.
Bình luận