Để hiểu rõ hơn về Spring:
- Spring phần 1: Spring là gì ? Giới thiệu Spring Framework trong Java
- Spring phần 2: Cài đặt Spring Tool Suite Cho Eclipse
Và cùng đến với Series Spring Core:
- Spring Core – Phần 1: Spring IoC , Inversion of Control trong Spring
- Spring Core – Phần 2: Spring Bean, Các scope trong Spring, Spring Bean Scope
- Spring Core – Phần 3: Spring Dependency Injection, DI trong Spring, so sánh CI – SI
- Spring Core – Phần 4: Spring Dependency Injection với Object, Collections, Map
- Spring Core – Phần 5: Spring AOP là gì? code ví dụ với Spring AOP
- Spring Core – Phần 6: AspectJ là gì? Spring AOP + AspectJ ví dụ với AspectJ
- Spring Core: Phần 7 – Spring PropertyPlaceholderConfigurer, lấy dữ liệu từ file properties
- Spring Core – Phần 8: Autowiring trong Spring, annotation @Autowired trong Spring, các kiểu autowiring
- Spring Core – Phần 9: Spring Auto Component Scanning, Các annotation hay dùng trong Spring
- Code ví dụ Spring đọc file từ resource folder (resources)
- Code ví dụ gửi email – gmail với Spring
1. Spring AOP là gì?
Aspect Oriented Programming (AOP) là 1 kỹ thuật lập trình dùng để tách logic chương trình thành các phần riêng biệt…
Trong Spring AOP, có 4 loại advice được hỗ trợ:
- Before advice: chạy trước khi method được thực thi
- After returning advice: Chạy sau khi method trả về một kết quả
- After throwing adivce: Chạy khi method ném ra một exception
- Around advice: Chạy khi method được thực thi (Bao gồm cả 3 loại advice trên)
Ở bài trước mình đã giới thiệu rõ AOP là gì, tác dụng và đặc điểm của nó nên trong bài này mình sẽ chủ yếu tập trung vào cách thực thi AOP trong Spring.
2. Code ví dụ với Spring AOP.
Nhắc lại một chút về một số khái nhiệm và thuật ngữ trong AOP với Spring (Phần này hơi trừu tượng một chút, các bạn đối chiếu với ví dụ bên dưới để hiểu hơn)
- Join point: là các điểm trong chương trình ví dụ điểm thực thi method (method execution), điểm xử lý excpetion, field access… Spring chỉ hỗ trợ method execution join point
- Advice: một hành động thực hiện ở joint point
- Pointcut: Là expression language giúp khớp nối với joint point
- Introduction: Cho phép introduce các new interface tới bất kì object adviced nào.
- Target Object: Object sẽ được adviced
- Aspect: là một class bao gồm các advice và các joint point
- Interceptor: là một aspect chỉ có duy nhất một advice
- AOP Proxy: dùng để cài đặt các Aspect
- Weaving: là tiến trình nối các aspect với các object, types để tạo nên advised object.
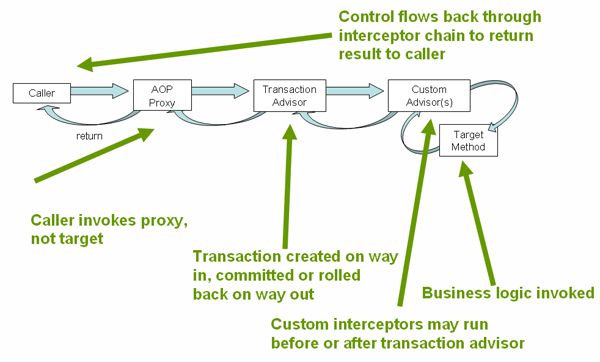
Ví dụ kinh điển với AOP mà ta hay dùng đó là chương trình thực hiện log.
Bây giờ mình sẽ tạo 1 class với các method, sau đó áp dụng AOP để thực hiện log các method của class theo cả 4 loại advice:
Khai báo các thư viện để sử dụng applicationContext, bean, aop trong spring:
<!-- https://mvnrepository.com/artifact/org.springframework/spring-beans -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-beans</artifactId>
<version>4.3.13.RELEASE</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.springframework/spring-context -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>4.3.13.RELEASE</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.springframework/spring-aop -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-aop</artifactId>
<version>4.3.13.RELEASE</version>
</dependency>
Tạo class Hello.java với 3 method:
public class Hello {
public void method1() {
System.out.println("+++++++++++++++++++++++++++++++");
System.out.println("method 1");
}
public void method2() {
System.out.println("+++++++++++++++++++++++++++++++");
System.out.println("method 2");
}
public void method3() {
System.out.println("+++++++++++++++++++++++++++++++");
System.out.println("method 3");
throw new IllegalArgumentException();
}
}
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd">
<bean id="hello" class="stackjava.com.springaop.Hello" />
</beans>
Demo:
public static void main(String[] args) throws Exception {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
Hello hello = (Hello) context.getBean("hello");
hello.method1();
hello.method2();
}
Kết quả:
+++++++++++++++++++++++++++++++
method 1
+++++++++++++++++++++++++++++++
method 2
Áp dụng before advice
Tạo class DemoBeforeAdvice.java định nghĩa hành động trước khi cách method của class Hello được chạy:
public class DemoBeforeAdvice implements MethodBeforeAdvice {
public void before(Method method, Object[] args, Object target) throws Throwable {
System.out.println("before method: " + method.getName());
}
}
MethodBeforeAdvice: là một aspect đồng thời cũng là một Interceptor vì nó chỉ có 1 advice
DemoBeforeService: là một AOP proxy, nó cài đặt lại aspect là MethodBeforeAdvice
aplicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd">
<bean id="hello" class="stackjava.com.springaop.Hello" />
<bean id="demoBeforeAdvice" class="stackjava.com.springaop.DemoBeforeAdvice" />
<bean id="helloProxy" class="org.springframework.aop.framework.ProxyFactoryBean">
<property name="target" ref="hello" />
<property name="interceptorNames">
<list>
<value>demoBeforeAdvice</value>
</list>
</property>
</bean>
</beans>
target object sẽ là đối tượng hello.
Demo:
public static void main(String[] args) throws Exception {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
Hello hello = (Hello) context.getBean("helloProxy");
hello.method1();
hello.method2();
}
Kết quả:
before method: method1
+++++++++++++++++++++++++++++++
method 1
before method: method2
+++++++++++++++++++++++++++++++
method 2
Tương tự mình sẽ tạo các proxy thực hiện cài đặt After returning advice, After throwing adivce, Around advice:
import java.lang.reflect.Method;
import org.springframework.aop.AfterReturningAdvice;
public class DemoAfterAdvice implements AfterReturningAdvice {
public void afterReturning(Object returnValue, Method method, Object[] args, Object target) throws Throwable {
System.out.println("after method: " + method.getName());
}
}
import org.springframework.aop.ThrowsAdvice;
public class DemoThrowAdvice implements ThrowsAdvice {
public void afterThrowing(IllegalArgumentException e) throws Throwable {
System.out.println("throw advice method: " );
}
}
import org.aopalliance.intercept.MethodInterceptor;
import org.aopalliance.intercept.MethodInvocation;
public class DemoAroundAdvice implements MethodInterceptor {
public Object invoke(MethodInvocation invocation) throws Throwable {
// same with MethodBeforeAdvice
System.out.println("around - before method: " + invocation.getMethod().getName());
try {
// proceed to original method call
Object result = invocation.proceed();
// same with AfterReturningAdvice
System.out.println("around - before method: " + invocation.getMethod().getName());
return result;
} catch (IllegalArgumentException e) {
// same with ThrowsAdvice
System.out.println("around - throw advice method: " + invocation.getMethod().getName());
throw e;
}
}
}
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd">
<bean id="hello" class="stackjava.com.springaop.Hello" />
<bean id="demoBeforeAdvice" class="stackjava.com.springaop.DemoBeforeAdvice" />
<bean id="demoAfterAdvice" class="stackjava.com.springaop.DemoAfterAdvice" />
<bean id="demoThrowAdvice" class="stackjava.com.springaop.DemoThrowAdvice" />
<bean id="demoAroundAdvice" class="stackjava.com.springaop.DemoAroundAdvice" />
<bean id="helloProxy" class="org.springframework.aop.framework.ProxyFactoryBean">
<property name="target" ref="hello" />
<property name="interceptorNames">
<list>
<value>demoBeforeAdvice</value>
<value>demoAfterAdvice</value>
<value>demoThrowAdvice</value>
<value>demoAroundAdvice</value>
</list>
</property>
</bean>
</beans>
Demo:
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class MainApp {
public static void main(String[] args) throws Exception {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
Hello hello = (Hello) context.getBean("helloProxy");
hello.method1();
System.out.println("\n");
hello.method2();
System.out.println("\n");
hello.method3();
}
}
Kết quả:
before method: method1
around - before method: method1
+++++++++++++++++++++++++++++++
method 1
around - before method: method1
after method: method1
before method: method2
around - before method: method2
+++++++++++++++++++++++++++++++
method 2
around - before method: method2
after method: method2
before method: method3
around - before method: method3
+++++++++++++++++++++++++++++++
method 3
around - throw advice method: method3
throw advice method:
Exception in thread "main" java.lang.IllegalArgumentException
Okay, Done!
Các bạn có thể download code ví dụ trên tại đây
Loạt bài chủ đề Java trên trang stackjava.com bản quyền thuộc thầy Trần Hữu Cương. Bài viết đăng trên blog Techmaster được sự đồng ý của tác giả.
Thầy Trần Hữu Cương đã và đang tham gia giảng dạy tại Techmater khoá Lộ trình Java Spring Boot Full Stack
Link gốc bài viết tại đây.
Bình luận