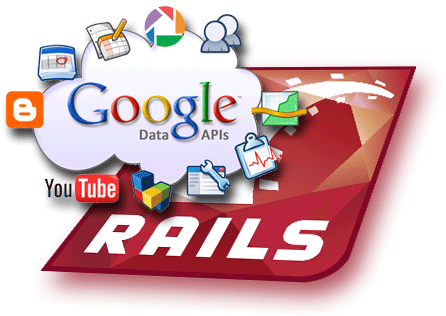
Chắc hẳn các bạn đều biết rằng Google và Facebook là hai ông lớn trong làng công nghê sở hữu một số lượng người dùng khổng lồ và cung cấp rất nhiều Apis để các ứng dụng khác có thể sử dụng và ‘ăn theo’. Đó là lí do mà bạn không thể không biết sử dụng API của họ. Ngày hôm nay, tôi sẽ hướng dẫn các bạn tích hợp Google Calendar Api vào dự án Rails thông qua sự hỗ trợ của browser.
Điều đáng nói là việc tích hợp Google API vào các dự án Ruby on Rails tương đối dễ dàng với một thư viện mà Google cung cấp sẵn cho các Ruby developers là google-api-ruby-client. Và tôi cũng sẽ sử dụng ứng dụng này để mọi chuyện trở nên dễ dàng hơn.
Go on!
Đầu tiên các bạn cần có một tài khoản Google. Và đương nhiên bạn cũng cần đăng kí một project mới tại https://console.developers.google.com/project
Sau đó, bạn hãy chắc chắn rằng mình có turn ON API đối với project mà bạn vừa tạo tại giao diện quản lý của project, chẳng hạn như Calendar API. Trong phần APIs & auth -> Credentials -> create new Client ID. Khi tạo new Client, bạn sẽ được đưa tới giao diện Consent screen, config email của bạn và product name là tên sản phẩm muốn sử dụng API của Google project này.
Sau khi tạo xong client, bạn sẽ có Client ID và Client Secret.
Đó là những công việc đầu tiên. Bạn có thể xem thêm tại https://developers.google.com/google-apps/calendar/firstapp, và https://developers.google.com/google-apps/calendar/v3/reference/
Tiếp theo, bạn có thể follow hướng dẫn tại thư viện google api ruby client và tham khảo các samples
Dưới đây là ví dụ nếu bạn chỉ xin quyền lấy dữ liệu từng user theo browser flow
require 'google/api_client'
require 'google/api_client/client_secrets'
require 'google/api_client/auth/installed_app'
# Initialize the client.
# application name là tên application mà bạn setup phía trên
client = Google::APIClient.new(
:application_name => 'Example Ruby application',
:application_version => '1.0.0'
)
## Yêu cầu user cấp quyền lấy dữ liệu từ API thông qua trình duyệt web
## Bạn có thể xem thêm các API trong samples của thư viện
plus = client.discovered_api('plus')
# Load client secrets from your client_secrets.json.
# client_secrets.json được download trên màn hình Google Developer Console
client_secrets = Google::APIClient::ClientSecrets.load
# Run installed application flow. Check the samples for a more
# complete example that saves the credentials between runs.
flow = Google::APIClient::InstalledAppFlow.new(
:client_id => client_secrets.client_id,
:client_secret => client_secrets.client_secret,
:scope => ['https://www.googleapis.com/auth/plus.me']
)
client.authorization = flow.authorize
# Make an API call.
result = client.execute(
:api_method => plus.activities.list,
:parameters => {'collection' => 'public', 'userId' => 'me'}
)
puts result.data
Còn nếu bạn sử dụng Google Service Account để tương tác giữa server và server, từ ứng dụng web của bạn và Google Cloud Storage chẳng hạn, bạn cần xác thực qua OAuth 2.0.
Đoạn code dưới đây sẽ giúp xác thực tài khoản Google Service của bạn.
key = Google::APIClient::KeyUtils.load_from_pkcs12('client.p12', 'notasecret')
client.authorization = Signet::OAuth2::Client.new(
:token_credential_uri => 'https://accounts.google.com/o/oauth2/token',
:audience => 'https://accounts.google.com/o/oauth2/token',
:scope => 'https://www.googleapis.com/auth/prediction',
:issuer => '123456-abcdef@developer.gserviceaccount.com',
:signing_key => key)
client.authorization.fetch_access_token!
client.execute(...)
Hope this can help you getting started with Google API!
Bình luận