Series Spring Boot: Spring Boot 0: Làm chủ Spring Boot, Zero to Hero và Series Spring Boot từ con số 0
Series Spring Core: tại đây
Dưới đây là Series Spring Thymeleaf
- Thymeleaf là gì? So sánh JSP, JSF với Thymeleaf
- Code ví dụ Spring MVC Thymeleaf Hello – dùng XML config
- Code ví dụ Spring MVC Thymeleaf Hello – dùng annotation config
- Submit form với Thymeleaf, Code ví dụ Spring Thymeleaf Form
- Code ví dụ hiển thị List, Set, Map với Thymeleaf
- Code ví dụ đa ngôn ngữ với Thymeleaf Internationalization / i18n
- Code ví dụ gửi email – gmail với Thymeleaf + Spring
Trong bài này mình sẽ thực hiện gửi email với định dạng html (bạn có thể tùy chỉnh hình ảnh, link, csss… trong email được gửi đi)
Code ví dụ gửi email – gmail với Thymeleaf + Spring
Tạo maven project:
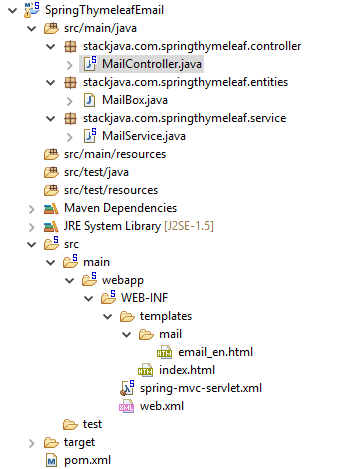
Thư viện sử dụng:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>stackjava.com</groupId>
<artifactId>SpringThymeleafEmail</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>war</packaging>
<properties>
<spring.version>5.0.2.RELEASE</spring.version>
<thymeleaf.version>3.0.9.RELEASE</thymeleaf.version>
</properties>
<dependencies>
<!-- Spring -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context-support</artifactId>
<version>${spring.version}</version>
</dependency>
<!-- Thymeleaf -->
<dependency>
<groupId>org.thymeleaf</groupId>
<artifactId>thymeleaf</artifactId>
<version>${thymeleaf.version}</version>
</dependency>
<dependency>
<groupId>org.thymeleaf</groupId>
<artifactId>thymeleaf-spring5</artifactId>
<version>${thymeleaf.version}</version>
</dependency>
<dependency>
<groupId>javax.mail</groupId>
<artifactId>mail</artifactId>
<version>1.4</version>
</dependency>
</dependencies>
</project>
File web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.5" xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd">
<display-name>SpringThymeleafEmail</display-name>
<servlet>
<servlet-name>spring-mvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>spring-mvc</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
</web-app>
File entities:
package stackjava.com.springthymeleaf.entities;
public class MailBox {
private String subject;
private String message;
private String recipientEmail;
// getter - setter
}
File Spring Config:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd">
<context:component-scan base-package="stackjava.com.springthymeleaf" />
<bean id="templateResolver"
class="org.thymeleaf.spring5.templateresolver.SpringResourceTemplateResolver">
<property name="prefix" value="/WEB-INF/templates/" />
<property name="suffix" value=".html" />
<property name="templateMode" value="HTML5" />
<property name="cacheable" value="false" />
</bean>
<bean id="templateEngine" class="org.thymeleaf.spring5.SpringTemplateEngine">
<property name="templateResolver" ref="templateResolver" />
</bean>
<bean class="org.thymeleaf.spring5.view.ThymeleafViewResolver">
<property name="templateEngine" ref="templateEngine" />
</bean>
<bean id="mailSender" class="org.springframework.mail.javamail.JavaMailSenderImpl">
<property name="host" value="smtp.gmail.com" />
<property name="username" value="teststackjava@gmail.com" />
<property name="password" value="stackjava123" />
<property name="javaMailProperties">
<props>
<prop key="mail.smtp.auth">true</prop>
<prop key="mail.smtp.port">587</prop>
<prop key="mail.smtp.starttls.enable">true</prop>
</props>
</property>
</bean>
</beans>
- Bean mailSender dùng để khai báo các thông tin cấu hình email dùng để gửi email (Xem lại: Gửi email – gmail với Spring)
- Tùy theo mail server mà bạn có cấu hình các thuộc tính cho mailSender khác nhau.
File Controller
package stackjava.com.springthymeleaf.controller;
import javax.mail.MessagingException;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import stackjava.com.springthymeleaf.entities.MailBox;
import stackjava.com.springthymeleaf.service.MailService;
@Controller
public class MailController {
@Autowired
MailService mailService;
@RequestMapping("/")
public String index(final Model model) throws MessagingException {
model.addAttribute("mailBox", new MailBox());
return "index";
}
@RequestMapping("/sendEmail")
public String sendEmail(@ModelAttribute("mailBox") MailBox mailBox, final Model model) throws MessagingException {
mailService.sendEmail(mailBox.getSubject(), mailBox.getMessage(), mailBox.getRecipientEmail());
return "redirect:/";
}
}
File Service
package stackjava.com.springthymeleaf.service;
import java.util.Locale;
import javax.mail.MessagingException;
import javax.mail.internet.MimeMessage;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.i18n.LocaleContextHolder;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.mail.javamail.MimeMessageHelper;
import org.springframework.stereotype.Service;
import org.thymeleaf.TemplateEngine;
import org.thymeleaf.context.Context;
@Service
public class MailService {
@Autowired
JavaMailSender mailSender;
@Autowired
TemplateEngine templateEngine;
public void sendEmail(String subject, String message, String recipientEmail) throws MessagingException {
Locale locale = LocaleContextHolder.getLocale();
// Prepare the evaluation context
Context ctx = new Context(locale);
ctx.setVariable("message", message);
// Prepare message using a Spring helper
MimeMessage mimeMessage = mailSender.createMimeMessage();
MimeMessageHelper mimeMessageHelper = new MimeMessageHelper(mimeMessage, "UTF-8");
mimeMessageHelper.setSubject(subject);
mimeMessageHelper.setTo(recipientEmail);
// Create the HTML body using Thymeleaf
String htmlContent = "";
htmlContent = templateEngine.process("mail/email_en.html", ctx);
mimeMessageHelper.setText(htmlContent, true);
// Send email
mailSender.send(mimeMessage);
}
}
- Phần gửi email tương tự với gửi email sử dụng java, spring
- Tuy nhiên ở đây ta sử dụng templateEngine để đọc file html để lấy nội dung, và truyền các tham số vào nội dung html đó.
- Bạn có thể áp dụng đa ngôn ngữ khi email bằng cách tạo các file html với các ngôn ngữ khác nhau hoặc truyền các ngôn ngữ khác nhau qua tham truyền vào file html (Xem lại đa ngôn ngữ với Thymeleaf)
File view:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Spring MVC + Thymeleaf + Send email</title>
</head>
<body>
<h2>Thymeleaf - Send Email</h2>
<form th:action="@{/sendEmail}" th:object="${mailBox}" method="post">
<table>
<tr>
<td>To:</td>
<td><input th:field="*{recipientEmail}" /></td>
</tr>
<tr>
<td>Subject:</td>
<td><input th:field="*{subject}" /></td>
</tr>
<tr>
<td>Message:</td>
<td><textarea rows="5" cols="30" th:field="*{message}"></textarea>
</td>
</tr>
<tr>
<td><input type="submit" value="Submit" /></td>
</tr>
</table>
</form>
</body>
</html>
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8" />
</head>
<body>
<img alt="stackjava.com" height="50px" width="50px"
src="https://stackjava.com/wp-content/uploads/2017/11/cropped-cropped-icon-new-1.png"/>
<br/>
<a href="https://stackjava.com">https://stackjava.com</a>
<br/>
Demo send email using Spring + Thymeleaf:
<p th:text="${message}"></p>
</body>
</html>
- File email_en.html ở đây được mình sử dụng làm mẫu để gửi email có định dạng html
Demo:
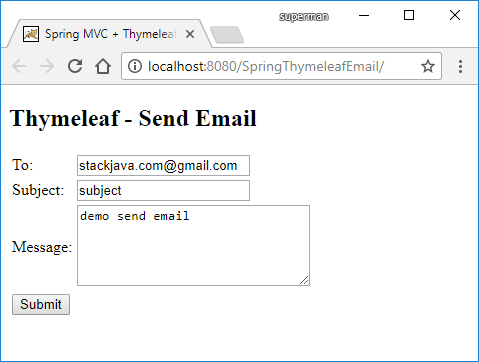
Kết quả:
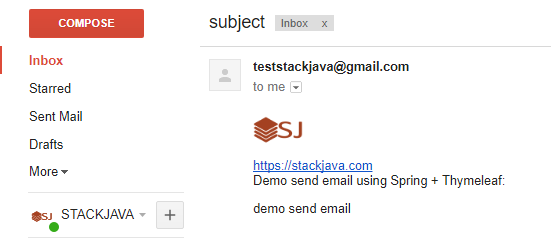
Các bạn có thể đọc lại bài Gửi gmail bằng Java để thực hiện code ví dụ gửi email với file đính kèm…
Okay, Done!
Download code ví dụ trên tại đây
Loạt bài chủ đề Java trên trang stackjava.com bản quyền thuộc thầy Trần Hữu Cương. Bài viết đăng trên blog Techmaster được sự đồng ý của tác giả.
Thầy Trần Hữu Cương đã và đang tham gia giảng dạy tại Techmater khoá Lộ trình Java Spring Boot Full Stack
Link gốc bài viết tại đây.
Bình luận